We will use Java as programming language. This project will try to cover the following requirements. We will try to first define entities and then services. We will also build class diagram as we proceed as I don’t want to bombard with a picture fill of random boxes and arrows at the end.
If you are new to java, this post will be a good start.
Requirement
- Register Rider
- Register driver
- Book a ride within the given radius
- Show ride history of the ride
- Update location of the cab
- Use In memory database
Design Motivation
Rider Entity
Rider Entity will have following fields:
- Name
- Phone Number
- Country Code
- List of booking Ids that this consumer has completed
Driver Entity
Driver entity should have following fields:
- Name
- Phone Number
- Country Code
Possibility of abstract class Personal Info
If we notice following fields are common in both the entities:
- Name
- Phone Number
- Country Code
That mean we can safely abstract out a PersonalInfo class which will be extended by both Driver and Rider class. Rider class can have “List of booking Ids that this consumer has completed”
At this point, our class diagram should look like one below:
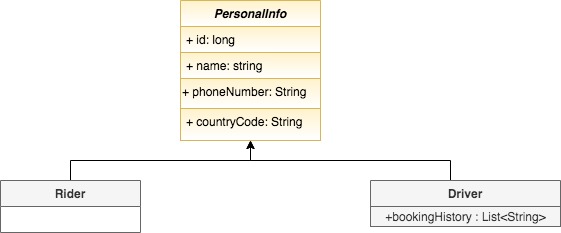
Vehicle Entity
Vehicle entity should have following fields:
- Car Number
- Latitude
- Longitude
- Type – To store type of car, for example: SUV, Compact, Sedan etcetera
- IsAvailable – To store if the car is available to hire or not.
- DriverId – We will not tightly couple driver and the vehicle. So, whenever a driver will login, he will pick up one of the vehicle. This field will store the id of driver who is currently driving this vehicle

Booking Entity
Booking entity should have following fields:
- Booking Id
- Rider User Id
- Car Number
- Start Time
- End Time
- Status
Storage Service
The requirement says that we need to use in-memory database. But what if tomorrow, someone asks us to implement the storage in database. Or in cache, something like Redis or memcache.
We will make an IStorageService. This will be an interface which will currently have just one implementation that stores data in-memory using Maps and Lists.
This interface will be like the one shown in gist below:
Our new class diagram should look like the one below:

Rider Service, Driver Service, Vehicle Service and Booking Service
The first three services will be again have interfaces to save the data of new rider, driver and vehicle. In addition to that, vehicle service should also have contract to find the vehicle near the given position within the given distance as well as a contract to update the position of vehicle.
These three different services will be injected with Storage Service at initialisation so as to store the data.
Booking Service will have Vehicle Service injected in addition to the Storage Service as during booking we need to find vehicles within the given radius.
If you don’t understand this statement right now, its okay. Please wait for the main class at the end. Hope it will be clear then.
The class diagrams looks like this now:

Download the project
Download the project using the following link.
https://github.com/rohitsingh20122992/cab
Main Class
Drop comment to discuss about the post.
If you liked this article and would like one such blog to land in your inbox every week, consider subscribing to our newsletter: https://skillcaptain.substack.com
the function bookingHistory() is a part of Rider Class and not Driver class.
LikeLiked by 1 person
Thanks and good catch!
LikeLike